How To Change An Item In A List Python
In this tutorial, you'll acquire how to use Python to supplant an item or items in a list. You'l learn how to supervene upon an detail at a detail index, how to replace a particular value, how to replace multiple values, and how to replace multiple values with multiple values.
Beingness able to work with lists is an of import skill for any Python programmer, given how prevalent and understandable these Python data structures are.
Let'southward get started with looking at what you'll learn!
Supercede an Item in a Python List at a Item Alphabetize
Python lists are ordered, meaning that we tin access (and modify) items when we know their index position. Python listing indices start at 0 and get all the style to the length of the listing minus ane.
Y'all can also access items from their negative index. The negative index begins at -ane
for the last particular and goes from there. To learn more than about Python listing indexing, check out my in-depth overview here.
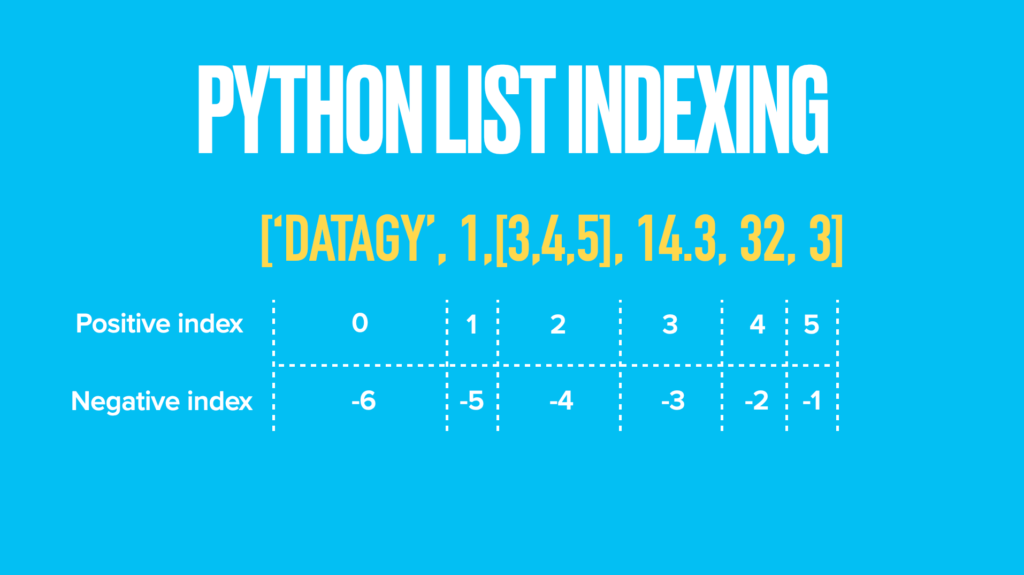
If we want to supplant a list detail at a item index, we can simply direct assign new values to those indices.
Let'south accept a await at what that looks like:
# Replace an item at a item index in a Python listing a_list = [ane, 2, three, 4, 5, 6, seven, viii, 9] # Mofidy get-go detail a_list[0] = 10 impress(a_list) # Returns: [x, 2, iii, iv, 5, 6, 7, eight, 9] # Change last item a_list[-1] = 99 print(a_list) # Returns: [x, ii, 3, four, five, vi, 7, 8, 99]
In the side by side department, you'll learn how to replace a item value in a Python list using a for loop.
Want to larn how to utilise the Python zippo()
function to iterate over two lists? This tutorial teaches you exactly what the zippo()
office does and shows you some creative means to use the function.
Supplant a Particular Value in a List in Python Using a For Loop
Python lists allow us to easily also change particular values. One style that we can do this is by using a for loop.
Ane of the primal attributes of Python lists is that they can contain duplicate values. Because of this, we can loop over each item in the list and check its value. If the value is one nosotros desire to supercede, then nosotros supervene upon it.
Permit's meet what this looks similar. In our list, the word apple
is misspelled. We want to supercede the misspelled version with the corrected version.
# Replace a particular item in a Python list a_list = ['aple', 'orangish', 'aple', 'banana', 'grape', 'aple'] for i in range(len(a_list)): if a_list[i] == 'aple': a_list[i] = 'apple' print(a_list) # Returns: ['apple', 'orange', 'apple tree', 'banana', 'grape', 'apple']
Let'south take a look at what nosotros've done here:
- We loop over each alphabetize in the list
- If the index position of the listing is equal to the item we want to replace, we re-assign its value
In the adjacent department, you'll learn how to plow this for loop into a Python list comprehension.
Desire to learn more near Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Desire to picket a video instead? Check out my YouTube tutorial hither.
Replace a Item Value in a Listing in Python Using a Listing Comprehension
1 of the central attributes of Python list comprehensions is that nosotros can often plough for loops into much shorter comprehensions. Let's see how nosotros tin use a listing comprehension in Python to supercede an item in a listing.
Nosotros'll utilize the aforementioned example we used in the for loop, to help demonstrate how elegant a Python list comprehension can exist:
# Replace a detail item in a Python list using a listing comprehension a_list = ['aple', 'orangish', 'aple', 'assistant', 'grape', 'aple'] a_list = ['apple' if item == 'aple' else item for item in a_list] print(a_list) # Returns: ['apple', 'orangish', 'apple', 'assistant', 'grape', 'apple']
We tin see hither that there are two main benefits of list comprehensions compared to for loops:
- Nosotros don't demand to initialize an empty listing
- The comprehension reads in a relatively plain English
In the next department, you'll larn how to modify all values in a listing using a formula.
Want to learn more than well-nigh Python list comprehensions? Bank check out this in-depth tutorial that covers off everything you lot need to know, with hands-on examples. More of a visual learner, check out my YouTube tutorial hither.
Alter All Values in a Listing in Python Using a Role
Neither of the approaches above are immediately articulate every bit to what they are doing. Because of this, developing a formula to practice this is a helpful approach to aid readers of your lawmaking understand what information technology is you're doing.
Let's take a look at how we tin use the listing comprehension approach and plough it into a formula:
# Replace a particular item in a Python list using a function a_list = ['aple', 'orange', 'aple', 'banana', 'grape', 'aple'] def replace_values(list_to_replace, item_to_replace, item_to_replace_with): return [item_to_replace_with if item == item_to_replace else detail for item in list_to_replace] replaced_list = replace_values(a_list, 'aple', 'apple') print(replaced_list) # Returns: ['apple', 'orangish', 'apple', 'assistant', 'grape', 'apple tree']
Here, we merely need to pass in the list, the particular we want to replace, and the item we desire to replace information technology with. The function name makes information technology easy to understand what we're doing, guiding our readers to better understand our actions.
In the next section, you'll acquire how to supercede multiple values in a Python list.
Want to larn how to use the Python zip()
function to iterate over two lists? This tutorial teaches y'all exactly what the zip()
office does and shows you some artistic ways to use the function.
Replace Multiple Values in a Python List
There may be many times when you want to replace not just a unmarried particular, but multiple items. This can be washed quite simply using the for loop method shown earlier.
Allow's take a expect at an example where we want to replace all known typos in a list with the give-and-take typo
.
# Replace multiple items in a Python list with the aforementioned value a_list = ['aple', 'ornge', 'aple', 'banana', 'grape', 'aple'] for i in range(len(a_list)): if a_list[i] in ['aple', 'ornge']: a_list[i] = 'typo' print(a_list) # Returns: ['typo', 'typo', 'typo', 'banana', 'grape', 'typo']
Similar to the for loop method shown earlier, we check whether or not an item is a typo or not and replace its value.
In the next section, you'll learn how to replace multiple values in a Python list with different values.
Check out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas!
Replace Multiple Values with Multiple Values in a Python List
The approach in a higher place is helpful if we want to replace multiple values with the same value. There may be times where you desire to replace multiple values with different values.
Looking again at our example, we may want to replace all instances of our typos with their corrected spelling. We tin once again apply a for loop to illustrate how to do this.
Instead of using a unmarried if
statement, we'll nest in some elif
statements that check for a value'due south value before replacing.
# Replace multiple items in a Python list with the different values a_list = ['aple', 'ornge', 'aple', 'banana', 'grape', 'aple'] for i in range(len(a_list)): if a_list[i] == 'aple': a_list[i] = 'apple' elif a_list[i] == 'ornge': a_list[i] = 'orange' print(a_list) # Returns: ['apple tree', 'orange', 'apple', 'assistant', 'grape', 'apple tree']
Demand to check if a primal exists in a Python dictionary? Cheque out this tutorial, which teaches yous v unlike ways of seeing if a primal exists in a Python dictionary, including how to render a default value.
Decision
In this tutorial, you learned how to use Python to supercede items in a listing. You learned how to use Python to supercede an item at a item index, how to supersede a particular value, how to modify all values in a listing, and how to supercede multiple values in a list.
To larn more than well-nigh Python list indexing, check out the official documentation here.
Source: https://datagy.io/python-replace-item-in-list/
Posted by: halseypeadlead.blogspot.com
0 Response to "How To Change An Item In A List Python"
Post a Comment